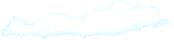
Interest breaks Capitalism
February 26th, 2024So, one way that I know, for sure, the US is in no way a Christian nation is that all over the place in the US, everywhere money is being loaned, interest is being charged. Jesus certainly never went off on the subjects of abortion or sex before marriage, but charging interest made him flip over some tables in a temple because he was so mad.
Jesus was right to be mad, because charging interest *breaks* capitalism. Here’s the basic problem. In our system, money is a pointer to value. The little bits of green paper have no actual value but you can use them to buy various goods, so they are backed by all they can buy.
When you charge interest, you are doing something with the pieces of paper which cannot be matched in the real world. Very few real world activities generate more actual value just by holding a certain amount of value – farming being the one exception. But, because you’re making the accounting system do something that doesn’t match reality, *trouble* ensues. We’ve talked about in previous episodes how the great depression was caused by a failure in the accounting system – and that’s the kind of failure you can run into.
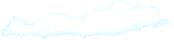
TascamSlurp
February 16th, 2024This is a perl script that can be used to pull all the files from a DA-6400 and automatically divide them into folders based on their timestamps
#!/usr/bin/perl
$|=1;$targetbase = “~/DownloadLocation”;
$host = “tascam”;use Net::FTP;
use Time::Local qw ( timelocal );use Data::Dumper;
print “Creating FTP object\n”;
$ftp = Net::FTP->new($host, Debug => 0) || die “Can’t connect to tascam”;
print “Logging in\n”;$ftp->login(“DA-6400″,”DA-6400”) || die “Can’t login ” , $ftp->message;
print “CWD\n”;
$ftp->cwd(“/ssd/DA Files”) || die “Cannot CWD: ” . $ftp->message;
print “BIN\n”;
$ftp->binary() || die “Cannot set to bin mode: ” . $ftp->message;my $list = $ftp->ls();
my $maxtime = 0;
my $timestamps = {};# determine newest date
foreach $file (@{$list}) {
next if($file eq “.”);
next if($file eq “..”);
next if(!($file =~ /.*.wav/));my $unixtime = getFileTimestamp($file);
my $ts = getTimestamp($unixtime);print “file: [$file] ts: $ts\n”;
$maxtime = $unixtime if($unixtime > $maxtime);
$timestamps->{$unixtime} = 1;
}
foreach $ts (keys %{$timestamps}) {$targetstub = getTargetDir($ts);
$targetdir = $targetbase . ‘/’ . $targetstub;
if(! -d $targetdir) {
mkdir $targetdir;
} else {
next;
}$targetdir .= “/ftp”;
if(! -d $targetdir) {
mkdir $targetdir;
}chdir $targetdir;
print “$ts – Writing to $targetdir\n”;foreach $file (@{$list}) {
next if($file eq “.”);
next if($file eq “..”);
next if(!($file =~ /.*.wav/));my $unixtime = getFileTimestamp($file);
if($unixtime == $ts) {
print “Fetching $file ..”;
$ftp->get($file);
print (-s $file);
print “\n”;
}
}
}sub getTargetDir
{
my $time = shift;
my ($sec,$min,$hour,$mday,$mon,$year,$wday,$yday,$isdst) = localtime($time);
return sprintf(“%02d%02d%04d”,$mon+1,$mday,$year+1900);
}sub getTimestamp
{
my $time = shift || time();my ($sec,$min,$hour,$mday,$mon,$year,$wday,$yday,$isdst) = localtime($time);
return sprintf(“%02d/%02d/%04d-%02d:%02d:%02d”,$mon+1,$mday,$year+1900,$hour,$min,$sec);
}sub getFileTimestamp
{
my $file = shift;
my ($prefix, $datetime, $take, $channel, $name) = split(/_/, $file);print “Datetime: [$datetime]\n” if($main::debug);
my ($date, $time) = split(/-/, $datetime);
print “Date: [$date]\n” if($main::debug);my ($yy, $MM, $dd) = $date =~ /(\d{4})(\d{2})(\d{2})/;
my ($hh, $mm, $ss) = $time =~ /(\d{2})(\d{2})(\d{2})/;print “yy: [$yy] mm: $mm dd: $dd\n” if($main::debug);
$MM -= 1;
$yy -= 1900;my $unixtime = timelocal($ss, $mm, $hh, $dd, $MM, $yy);
return $unixtime;
}
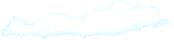
The boundaries between neural networks
February 15th, 2024So, I’ve been working on a theory.. this is more of my hand-wavy guessing what’s going on inside a NNN stuff..
My theory is that children grow their ego.. the portion of their decision trees that is recognizably them – from the inside out. At the same time, society grows it’s internal manifestation of state from the outside in. When combined with the fact that humans have a large number of neurons between their senses and their conscious experience – the part of them that is “on the ride” – there are some problems that can crop up here.
My mother had stated at one point that when I was younger she thought that I wanted to go to seminary school. I think what was actually going on there was she was seeing reflected light of her own beliefs through the upper layer because my ego had not yet grown the strength to manifest and clearly communicate my opinions about Christianity and my desires surrounding it.
Now, I’m going to segue for a second (I promise this is all related, at least in my mind) to say that I have nothing against people choosing to be transgendered. I have a number of friends who are, or who have experimented with it. I see nothing wrong with us choosing our gender identities and if it were up to me we would be able to quickly and easily change the configuration of our bodies to match, or even experiment with different alternatives. I would certainly be curious to experience being female. For them as argue that we are thwarting God’s will, I would respond that our bodies are gifts and when I give a gift I don’t give it with the expectation that the receiver will not modify it or re-gift it if it doesn’t work out for them. I might be sad that they choose to do so but I certainly would not feel that I should be in a position to force them to do otherwise or that it is a sin.
The reason I bring all this up, though, is I know of at least one case in which a child did not want to be transgendered but it appeared at least from the outside that they did. And I think it’s important to understand the reflected light phenomenon and be aware of the fact that – in cases like religion and gender identity – one can’t really tell with young children what they want because they will have a significant challenge in communicating that when the society-programmed layers nearer their senses will tend to conform to whatever the visible authority figures in their lives are pushing.
I think this is a big problem in the case of religion because religions are viral – most of them have survived by being informational virii after all – which is why people should not force their children to go to church or be confirmed in a specific religion. Removing this informational virus after it has been installed is basically impossible – NNNs have no delete – and it can only be disabled after considerable effort. I do not think I have yet removed all the deleterious effects of Christianity, for example.
In the case of people changing gender identity, all indications from my own personal exposure to the result are it can be even more traumatic. What I’m saying is, there’s time – children don’t really need to have a specific gender identity and I see no problem with them wearing the clothing and having the pronouns of either gender but one should definitely make absolutely and very sure that they are really onboard, for themselves, before using hormones or having surgery. (I think the latter is not a problem because I don’t think confirmation surgery is done until after puberty). When I was first told of one individual choosing to be a confirmed transgender who was very young my response was I wasn’t really sure how you could possibly know until after puberty, although I understand that on the other side of that is that it gets a lot harder post puberty and so puberty blockers are a time-sensitive thing.
I don’t know. In most cases I freely admit transgender issues are well beyond my pay grade and my opinions mean very little. But I do think that *in general* psychologists and psychiatrists should be aware of what is actually happening in the neural network and aware of the possibility of confirmation bias on the part of the caregivers for children, and the possibility that children are not able to communicate their true needs and desires because they are still very deep inside their minds and slowly learning how to reach the outside and when and how to push back against authority.
(As a side note, I do think our society is somewhat aware at least subconsciously of the problem here and it is why one should absolutely not participate in sex with children. We understand that they can’t give informed consent for sex and that they might not speak of their true needs because of the power imbalance issue. I think we just don’t always realize that this problem is prevalent all over the place)
Our children are not our property – to the extent they belong to anyone they belong to themselves and to the tribe as a whole. Forcing certain types of decisions is likely to have a bad effect on them and a bad effect on all of us in the sense that we are all connected.
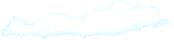
Braces
January 21st, 2024How anyone can look at their kids needing braces and not realize we’re not the result of direct engineering is beyond me.
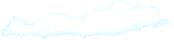
Hell
January 2nd, 2024My theory is the only person who deserves eternal punishment is anyone who would sentance someone else to eternal punishment – and only until they repent. (Yes, I just put the Christian God in hell until He learns. 🙂 )
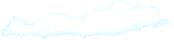
Nov1 – another mellow movie soundtrack track
November 1st, 2023For anyone who has been missing my mellow movie-soundtrack style tracks, here is one:
I am trying to get back into writing some more
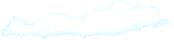
1000 hours
October 11th, 2023So, sometime early in COVID I installed a hour meter.. I’m not sure exactly when, but it hit 200 hours in Oct of 2020. Anyway, I always said I’d do a twitch show when it hit 1000 hours, and so here it is
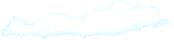
Love and Christianity
July 16th, 2023So, I have yet another disproof of Christianity. (I know, I know, I should really let go of this obsession – but a figure driven by Christianity still gatekeeps my dreams – a copy of my mother as she was when I was young – and I really have to get it and her entirely out of my mind, and I feel like coming up with arguments against it helps this process)
This one’s really simple, and is based on my experiences with $_PERSON.
If God loves us, Hell would also be a good place.
Love does not want the beloved to be unhappy if the beloved wants nothing to do with the one who loves. Love wants the beloved to be happy and healthy and have everything they want and need. Anything lesser is a misunderstanding of love. Part of what’s so upsetting about $_PERSON not wanting to talk to me is she might not be okay and I would never know and there would never be anything I could do to improve the situation. I cannot *fathom* a God of love choosing for someone to suffer because they didn’t want to be near said God. I also cannot fathom said God deleting such people, as some have suggested – the idea of $_PERSON ceasing to exist is inherently extremely painful.
To me, this is a obvious doomsday knell for Christianity. The one Christian I presented this too suggested God is goodness and that there can be no goodness without God. This shows a inadequate understanding of the data universe.
Good feelings, in us, come from good experiences. Love is inherently in our hardware so even if we are banished to another realm we are still going to love, and we are still going to try to give each other good experiences. Good experiences are *data*, and *God can’t make data become unavailable from the universe*. My example was God could decide to banish the number 2, but if a computer runs for($i=0;$i<10;$i++) $i is still going to contain 2 right after it contains 1. It's possible God could actively, via hostility, constantly erase such things from our memories, but there is no possible way you're going to claim that Loki-God is a God of Love.
In Anathem, Neil Stephenson speaks of a very important idea – he speaks of it as the Hylaean Theoretical World, or the HTW, and it’s where all the perfect abstract concepts live that we iterate through when we use our imaginations. It contains, for example, every possible set that can exist. Now, you can imagine a computer that has a portion that can work on countable infinities mated to a classical computer, and you can imagine that you could use such a computer to, for example, find and iterate through every data element that would be every possible hug from a particular person, for example. Of course, in the real world, we find these data members using much more clumsy but intuitive means, like hugging people, but the point is that the *experience* of a hug is a data experience to us – it’s a bunch of information being streamed to our brain from our body. And that dataset will be in hell – in fact if there are multiple Gods or multiple universes that data is in potentia available to all of them, because the HTW is *bigger than God*. It’s a different type of entity than God could ever be – and it’s certainly not all good. Those same datasets have every way you could ever be tortured, for example. But the point is, it’s not something God can make “go away” because it’s not a concrete manifestation. Just as God can’t change pi no matter how hard he tries, he can’t make any place lack goodness. So that argument also does not hold water.
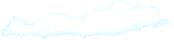
Using Device::Hue
June 11th, 2023So, I found a major lack of documentation for Device::Hue, and chatGPT had some information that I would describe as whimsically wrong.
Here’s what I ended up doing to get a working perl setup controlling my hue lights:
1) Put a valid URL in /etc/environment for the key HUE_BRIDGE
2) Put a key in /etc/environment for the key HUE_KEY
3) The following code sets lights 17 and 19 to red:
my $bridge = Device::Hue->new();
$bridge->config();
foreach $light_id (17, 19) {
my $light = $bridge->light($light_id);$light->set_state( { hue => 0, sat => 254 });
$light->commit();
}